mirror of
https://github.com/muerwre/vault-frontend.git
synced 2025-07-01 05:48:28 +07:00
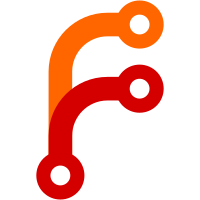
* added notes sidebar * added note dropping and editing * added sidebar navigation * handling sidebarchanges over time * using router back for closing sidebar * fixed tripping inside single sidebar * added superpowers toggle to sidebar * user button opens sidebar now * added profile cover for profile sidebar * removed profile sidebar completely * ran prettier over project * added note not found error literal
34 lines
921 B
TypeScript
34 lines
921 B
TypeScript
import { useCallback, useEffect } from "react";
|
|
|
|
import isBefore from "date-fns/isBefore";
|
|
|
|
import { useRandomPhrase } from "~/constants/phrases";
|
|
import { useLastSeenBoris } from "~/hooks/auth/useLastSeenBoris";
|
|
import { useBorisStats } from "~/hooks/boris/useBorisStats";
|
|
import { IComment } from "~/types";
|
|
|
|
export const useBoris = (comments: IComment[]) => {
|
|
const title = useRandomPhrase("BORIS_TITLE");
|
|
|
|
const { lastSeen, setLastSeen } = useLastSeenBoris();
|
|
|
|
useEffect(() => {
|
|
const last_comment = comments[0];
|
|
|
|
if (!last_comment) return;
|
|
|
|
if (
|
|
!last_comment.created_at ||
|
|
!lastSeen ||
|
|
isBefore(new Date(lastSeen), new Date(last_comment.created_at))
|
|
) {
|
|
return;
|
|
}
|
|
|
|
void setLastSeen(last_comment.created_at);
|
|
}, [lastSeen, setLastSeen, comments]);
|
|
|
|
const { stats, isLoading: isLoadingStats } = useBorisStats();
|
|
|
|
return { stats, title, isLoadingStats };
|
|
};
|