mirror of
https://github.com/muerwre/vault-frontend.git
synced 2025-04-24 20:36:40 +07:00
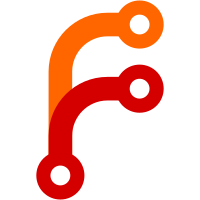
* fixed paths to match refactored backend * fixed some paths according to new backend * fixed auth urls for new endpoints * fixed urls * fixed error handling * fixes * fixed error handling on user form * fixed error handling on oauth * using fallback: true on node pages * type button for comment attach buttons * fixed return types of social delete * changed the way we upload user avatars
45 lines
1.1 KiB
TypeScript
45 lines
1.1 KiB
TypeScript
import React, { FC, useCallback } from 'react';
|
|
|
|
import { Button } from '~/components/input/Button';
|
|
import { ButtonGroup } from '~/components/input/ButtonGroup';
|
|
import { COMMENT_FILE_TYPES } from '~/constants/uploads';
|
|
|
|
interface IProps {
|
|
onUpload: (files: File[]) => void;
|
|
}
|
|
|
|
const CommentFormAttachButtons: FC<IProps> = ({ onUpload }) => {
|
|
const onInputChange = useCallback(
|
|
(event) => {
|
|
event.preventDefault();
|
|
|
|
const files = Array.from(event.target?.files as File[]).filter(
|
|
(file: File) => COMMENT_FILE_TYPES.includes(file.type),
|
|
);
|
|
if (!files || !files.length) return;
|
|
|
|
onUpload(files);
|
|
},
|
|
[onUpload],
|
|
);
|
|
|
|
return (
|
|
<ButtonGroup>
|
|
<Button iconLeft="photo" size="small" color="gray" iconOnly type="button">
|
|
<input type="file" onInput={onInputChange} multiple accept="image/*" />
|
|
</Button>
|
|
|
|
<Button
|
|
iconRight="audio"
|
|
size="small"
|
|
color="gray"
|
|
iconOnly
|
|
type="button"
|
|
>
|
|
<input type="file" onInput={onInputChange} multiple accept="audio/*" />
|
|
</Button>
|
|
</ButtonGroup>
|
|
);
|
|
};
|
|
|
|
export { CommentFormAttachButtons };
|